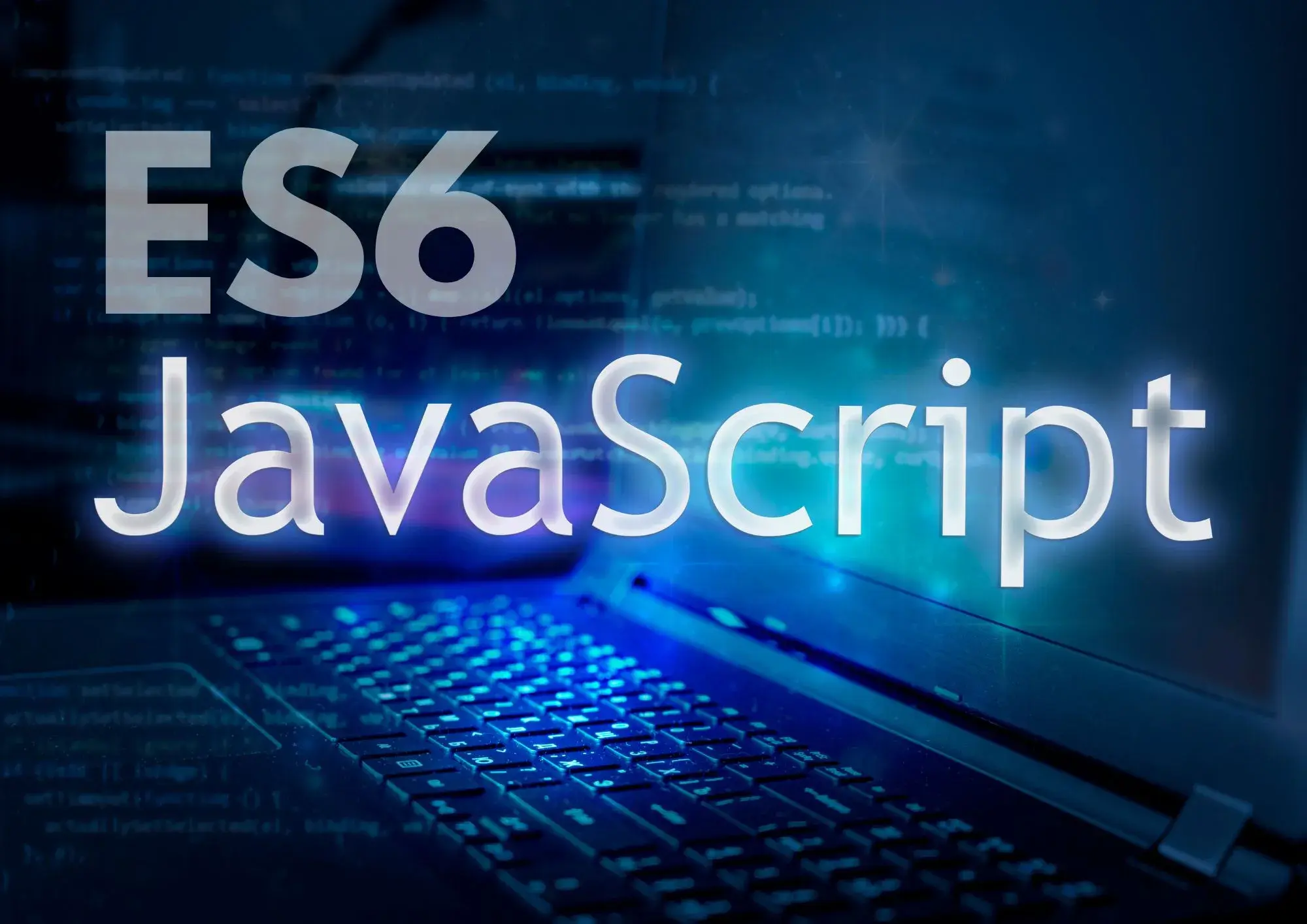
ES6 JavaScript Interview Questions: Exploring the Modern Features
ES6 (ECMAScript 2015) stands as a beacon of innovation, introducing a plethora of features that have redefined how developers write and understand JavaScript.
To truly grasp the depth and breadth of ES6, one must not only comprehend its syntax but also appreciate the subtleties and intricacies that these features bring to the table.
This collection of interview questions has been meticulously crafted to challenge even the most seasoned JavaScript developers, probing deep into the mechanics of ES6 and testing the limits of their understanding.
From the elegance of arrow functions and the expressiveness of template literals to the robustness of new asynchronous patterns, these questions will navigate through the nuanced intricacies of ES6, offering a comprehensive exploration of its most profound concepts.
1: What are arrow functions in ES6, and how do they differ from traditional functions?
Answer: Arrow functions are a concise way to write function expressions in ES6. They differ from traditional functions in that they do not have their own ‘this’, ‘arguments’, ‘super’, or ‘new.target’. They're best suited for non-method functions and offer a shorter syntax.
2. How do template literals in ES6 enhance string manipulation?
Answer: Template literals provide a more readable and concise syntax for creating strings. They allow for the embedding of expressions within strings, making it easier to construct strings dynamically. Template literals also support multi-line strings without the need for concatenation.
3. Can you explain destructuring in ES6?
Answer: Destructuring in ES6 provides a syntax for unpacking values from arrays or properties from objects into distinct variables. It simplifies the process of assigning properties of an object or elements of an array to separate variables.
4.What are default parameters, rest parameters, and the spread operator in ES6?
- Default parameters allow named parameters to be initialized with default values if no value or ‘undefined’ is passed.
function greet(name = 'Guest', greeting =
'Hello') {
console. log( `${greeting},
${name}!`);
}
// Calling the function without providing any parameters
greet(); // Output: Hello, Guest!
// Calling the function with only one parameter
greet('Alice'); // Output: Hello, Alice!
// Calling the function with both parameters
greet('Bob',
'Hi'); // Output: Hi, Bob!
- Rest parameters represent an indefinite number of arguments as an array, improving the handling of parameter lists.
function sum(...numbers) {
return numbers.reducereduce((total, num) => total + num, 0);
}
// Calling the function with different numbers of arguments
console.log(sum(1,
2, 3)); // Output: 6
console.log(sum(4,
5, 6, 7, 8)); // Output: 30
console.log(sum(10)); // Output: 10
console.log(sum());
- The spread operator allows an iterable (like an array) to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected.
// Example 1: Using spread in array literals
const numbers = [1, 2, 3];
const moreNumbers = [...numbers,4, 5, 6 ];
console. log(moreNumbers); // Output: [1, 2, 3, 4, 5,
6]
// Example 2: Using spread in function calls
function addNumbers(a, b, c) {
return a + b + c;
}
const values = [1, 2, 3];
const sum = addNumbers(...values);
console.log(sum); // Output: 6
5.What are the differences between ‘var’, ‘let’, and ‘const’ in ES6?
Answer: ‘var’ declares a variable globally or locally to an entire function regardless of block scope.
‘let’ allows you to declare variables that are limited to the scope of a block statement.
‘const’ is similar to ‘let’ but the variable declared must be immediately initialized, with the value remaining constant in its scope.
6.How do classes in ES6 improve the object-oriented programming paradigm in JavaScript?
Answer: Classes in ES6 provide a clearer and more concise syntax to create objects and handle inheritance. They make the code more structured and maintainable by offering a familiar way to define constructor functions and prototypes.
7. Can you explain how ES6 modules differ from the module pattern in JavaScript?
Answer: ES6 modules allow for the static analysis of dependencies. They support static import and export, which makes the structure of code more consistent and the dependencies clearer. Unlike the module pattern which encapsulates code, ES6 modules improve code organization and reusability without the complexity of closures.
8. How do promises improve asynchronous programming in JavaScript?
Answer: Promises in ES6 provide a cleaner and more robust way to handle asynchronous operations compared to traditional callback-based approaches. They allow you to attach callbacks to handle the fulfillment and rejection of asynchronous operations, making the code more readable and maintainable.
9. How does the temporal dead zone impact the behavior of ‘let’ and ‘const’ in ES6?
Answer: The temporal dead zone (TDZ) refers to the period between entering the block where the variable is defined and the actual declaration of the variable. During this time, accessing the variable will result in a ReferenceError. This behavior is significant for ‘let’ and ‘const’ declarations, as it helps in identifying bugs where a variable is used before its declaration, ensuring that the code is more predictable and less prone to runtime errors.
10. Can you explain the significance of the ‘Symbol’ primitive type introduced in ES6, and how it can be used to create private object members?
Answer: ‘Symbol’ is a new primitive type in ES6 that represents a unique and immutable value and can be used as the key of an Object property. In terms of creating private object members, Symbols can be used as property keys that are not enumerable in for...in loops, nor are they listed by ‘Object.keys()’ or ‘Object.getOwnPropertyNames()’, which makes them a good fit for private or internal object state that should not be accessible directly from outside the object.
11. Discuss the differences between Map and Object in ES6, and where would you prefer Map over Object?
Answer: A ‘Map’ holds key-value pairs where the keys can be of any datatype and maintains the order of elements. Unlike an object, keys in Map are not converted to strings, which allows for better performance in scenarios involving frequent addition and removal of key-value pairs. ‘Map’ is preferred over ‘Object’ when the keys are unknown until runtime, the keys are not strings, or when there's a need to maintain the insertion order of the keys.
12. How do Proxies in ES6 allow you to control the behavior of fundamental operations on objects? Give an example.
Answer: Proxies in ES6 allow you to define custom behavior for fundamental operations on objects (like property lookup, assignment, enumeration, function invocation, etc.). A ‘Proxy’ is created with two parameters: target (the original object) and handler (an object that defines which operations will be intercepted and how to redefine intercepted operations). For example, you can intercept operations like ‘get’, ‘set’, and ‘apply’ to log access to properties or validate the new value of properties.
13. Explain the concept of Tail Call Optimization (TCO) in ES6. How does it improve the performance of recursive functions?
Answer: Tail Call Optimization (TCO) in ES6 is a feature where the stack frame of a function is cleared if the last action of the function is a return call to another function. This optimization is significant in the context of recursive functions as it prevents creating a new stack frame for every call, thus saving memory and preventing stack overflow errors in deep recursion scenarios.
14. In ES6, can you explain the difference between iterable and iterator protocols? How do they work together in the context of a for...of loop?
Answer: The iterable protocol allows JavaScript objects to define or customize their iteration behavior. Meanwhile, the iterator protocol defines a standard way to produce a sequence of values (either finite or infinite). An object becomes iterable if it implements the @@iterator method, meaning the object (or one of the objects up its prototype chain) must have a property with a Symbol.iterator key. The for...of loop first calls the @@iterator method on the iterable to obtain an iterator. Then, it repeatedly calls the next() method on the iterator and iterates over the returned values until the done property is true.
// Implementing iterable and iterator protocols
constconst myIterable = {
data: [ 1, 2, 3],
[Symbol.iterator]: function () {
let index = 0;
return {
next: () => {
if (index < this.data.length) {
return {value : this.data[index++], done: false };
} else {
return { value: undefined, done:true };
}
}
};
}
};
// Using the for...of loop to iterate over the custom iterable
for (const value of myIterable) {
console. log(value);
}
Implement a memoization function in ES6.
15. Memoization is an optimization technique used to speed up function calls by caching the results of expensive function calls. Write a function memoize in ES6 that takes a function as an argument and returns a memoized version of the function.
const memoize
= (fn) => {
let cache = new Map();
return (...args) => {
let n = args[0]; // just taking one argument here
for simplicity
if (cache.has(n)) {
return cache.get(n);
}
let result = fn(n);
cache.set(n, result);
return result;
};
};
Create a custom iterable object in ES6.
16. In ES6, you can make an object iterable by using the Symbol.iterator property. Create an iterable object that iterates over the numbers 1 through 5.
const iterableObj = {
[Symbol.iterator]() {
let step = 0;
return {
next() {
step++;
if (step <= 5) {
return { value: step, done: false };
}
return { value: undefined, done: true };
}
};
}
};
for (const val of iterableObj) {
console.log(val); // Output: 1 2 3 4 5
}
Use the spread operator to merge two arrays and an additional element in ES6.
17. Write a function mergeArrays that takes two arrays and an additional element as arguments. The function should return a new array with the elements of the first array, the additional element, and then the elements of the second array, in that order.
const mergeArrays = (arr1, arr2, element) => {
return [...arr1, element, ...arr2];
};
const arr1 = [ 1, 2];
const arr2 = [ 4, 5];
console. log( mergeArrays(arr1, arr2, 3)); // Output: [1, 2, 3, 4, 5]
Implement a simple class in ES6 with a getter and a setter.
18. Define a class Person with private properties for the first and last name. Implement a getter and a setter for a fullName property, which should return or set the first and last names concatenated when accessed or assigned.
class Person
{
constructor(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
get fullName() {
return `${this.firstName} ${this.lastName}`;
}
set fullName(name) {
[this.firstName, this.lastName] = name.split(' ');
}
}
const person = new
Person('John', 'Doe');
console.log(person.fullName); // Output: John Doe
person.fullName = 'Jane Smith';
console.log(person.fullName); // Output: Jane Smith
Write an async function in ES6 that fetches data from a URL and logs the result.
19. Using ES6 features, write an async function named fetchData that takes a URL, uses the fetch API to get data from the URL, and logs the result. Ensure that the function properly handles exceptions.
const fetchData = async (url) => {
try {
const response = await fetch(url);
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching
data:', error);
}
};
20. Explain the concept of a higher-order function and provide an example of how it can be used in combination with array methods like map, reduce, or filter in ES6.
Answer: A higher-order function is a function that takes another function as an argument or returns a function as a result. It’s a core concept in functional programming. In ES6, higher-order functions are often used in combination with array methods. For example, map, reduce, and filter are higher-order functions because they take a function as an argument. This allows for powerful and concise data transformations. For instance, using map to square each number in an array: [1, 2, 3].map(n => n * n) results in [1, 4, 9].
21. Discuss how the use of promises and async/await in ES6 improves error handling in asynchronous code compared to traditional callback patterns..
Answer: Promises and async/await in ES6 offer a more robust way of handling asynchronous operations compared to traditional callback patterns. They allow for cleaner and more readable code. Promises represent the eventual completion (or failure) of an asynchronous operation and its resulting value. With async/await, you can write asynchronous code that looks synchronous, making it easier to understand and debug. It also simplifies error handling; instead of nested callbacks, you can use standard try/catch blocks.
22. Explain the concept of a generator function in ES6. How does it differ from a regular function, and what are its practical applications?
Answer: A generator function is a special type of function in ES6 that can pause and resume its execution. When called, it doesn’t run its code. Instead, it returns a special object called a generator object through which you control the execution of the generator function. Generators are useful for implementing lazy data structures, handling asynchronous calls more conveniently, and managing the flow of data through a series of steps. They are fundamentally different from regular functions in their ability to pause execution and yield multiple values over time, each time they are resumed.
23. In the context of ES6 modules, discuss the difference between named exports and default exports. What are the advantages and disadvantages of each, and in what scenarios would you use one over the other?
Answer: Named exports allow you to export multiple values from a module. When importing, you need to use the same name as the named export. Default exports let you export a single value from a module, which can be imported with any name. The advantage of named exports is that they explicitly specify what is being exported, making the code more readable and maintainable. However, they can be verbose. Default exports are useful when a module is designed to export a single main functionality. However, they can lead to inconsistencies in naming across different modules.
24. Illustrate how object property shorthand and method properties in ES6 can lead to cleaner and more concise object creation. Provide a comparison with pre-ES6 syntax.
Answer: In ES6, object property shorthand lets you create properties in an object, where the property name is the same as the variable name. Method properties allow you to define methods on an object without the function keyword. For example, pre-ES6, you would write const obj = {a: a, b: b, c: function() {}};. In ES6, you can write const obj = {a, b, c() {}};. This leads to cleaner and more concise code, especially in larger and more complex object structures.
Full Stack Development Courses in Different Cities
- Srinagar
- Bangalore
- Gujarat
- Haryana
- Punjab
- Delhi
- Chandigarh
- Maharashtra
- Tamil Nadu
- Telangana
- Ahmedabad
- Jaipur
- Indore
- Hyderabad
- Mumbai
- Agartala
- Agra
- Allahabad
- Amritsar
- Aurangabad
- Bhopal
- Bhubaneswar
- Chennai
- Coimbatore
- Dehradun
- Dhanbad
- Dharwad
- Faridabad
- Gandhinagar
- Ghaziabad
- Gurgaon
- Guwahati
- Gwalior
- Howrah
- Jabalpur
- Jammu
- Jodhpur
- Kanpur
- Kolkata
- Kota
- Lucknow
- Ludhiana
- Noida
- Patna
- Pondicherry
- Pune