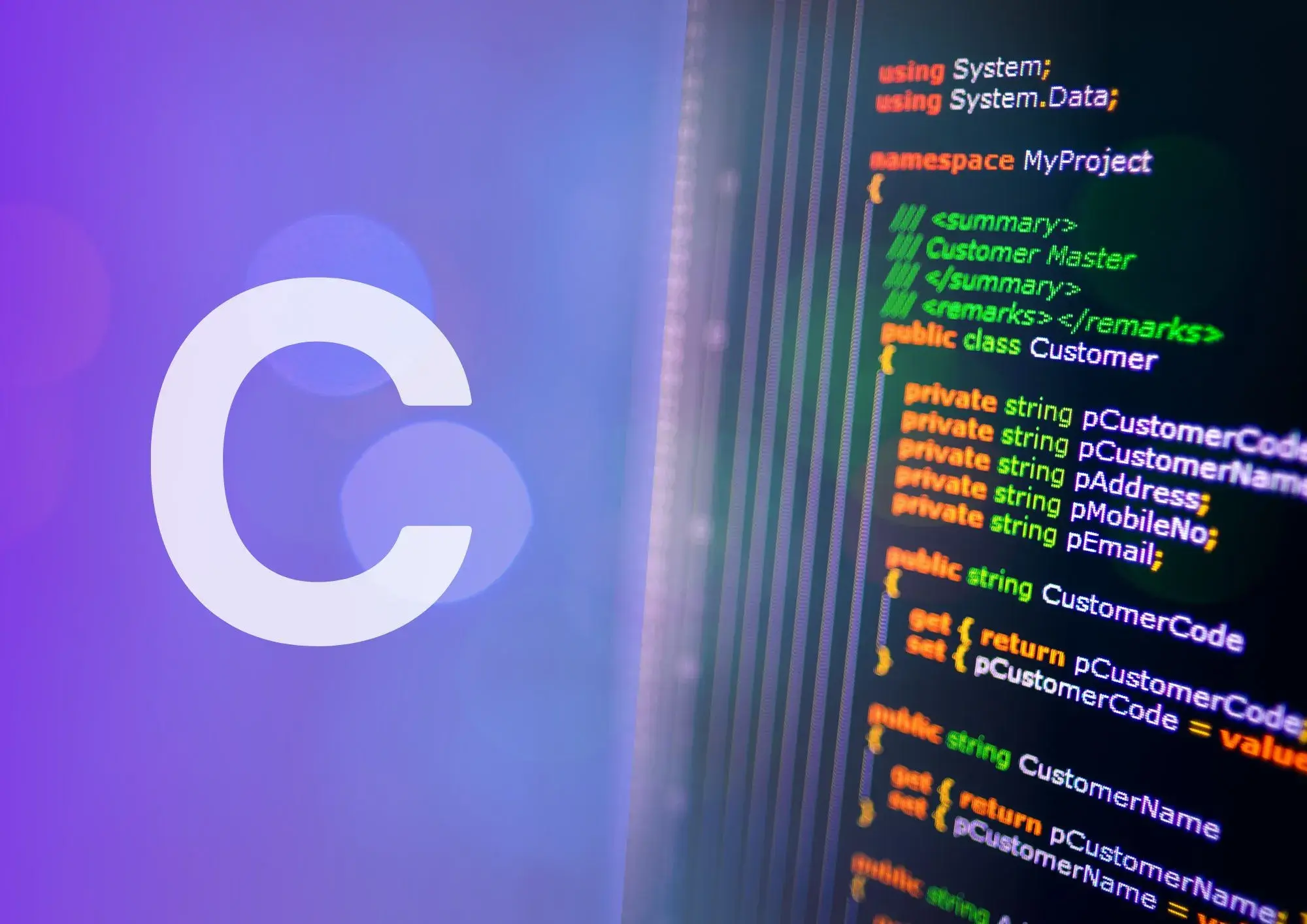
C Programming Tricky Questions
Introduction:
The C programming
language is still super popular, even though it's been around for a long time. A lot
of programmers, about 1 in 5, choose to use C because it's really good at
controlling computers closely. Because it's so useful, people who know C are likely
to get paid more in the coming years.
Interestingly, despite the surge in new languages, a substantial 75% of IoT (Internet of Things) developers opt for C, underscoring its critical role in shaping the future of smart technologies. These statistics not only underscore the enduring legacy of C but also point to a vibrant, continuously growing community of developers committed to harnessing its full potential.
Here are some of the C language tricky questions which every student should know.
Question1: How can you set a specific bit in a number using bitwise operators?
Answer: You can set the nth bit of a number num by num |= (1 << n).
Question2: What is the output of the following code snippet?
int arr[] = {10, 20, 30, 40, 50};
int *ptr1 = arr;
int *ptr2 = arr + 3;
printf (" %td ", ptr2 - ptr1);
Answer: The output will be 3 as the pointer difference gives the number of elements between the two pointers.
Question3: Why is memory alignment important in C programming, and how can it affect the performance of an application?
Answer: Memory alignment is crucial for performance optimization. Misaligned access can cause multiple memory accesses and cache misses, slowing down the application. It ensures that data is read in the most efficient way possible.
Question4: Explain how the volatile keyword impacts the optimization performed by the compiler with an example.
Answer: The volatile keyword tells the compiler that a variable's value may change at any time without any action being taken by the code. This prevents the compiler from optimizing the code in a way that assumes the value of the volatile variable remains constant.
Question5: What is the difference between static and extern storage class specifiers in C?
Answer: static limits the scope of a variable to its current file, preserving its value between function calls, while extern extends the visibility of a variable or function to the entire program.
Question6: Give an example of undefined behavior in C.
Answer: An example of undefined behavior is modifying a string literal. For instance, attempting char *str = "hello"; str[0] = 'H'; can lead to undefined behavior.
Question7: How do you declare and use a function pointer in C?
Answer: A function pointer is declared as return_type (*ptr_name)(parameter_types). You can use it to call a function by assigning the function to the pointer ptr_name = &function_name; and then calling it as (*ptr_name)(parameters);.
Question8: What is struct padding, and how can #pragma pack be used to control it?
Answer: Struct padding adds extra bytes to match memory alignment boundaries. #pragma pack(n) can be used to change the alignment to n bytes, minimizing the struct size and potentially impacting performance due to misalignment.
Question9: What are the common errors programmers make when using dynamic memory allocation in C?
Answer: Common errors include not checking the return value of malloc or calloc (which may return NULL), memory leaks (not freeing memory), and dangling pointers (accessing freed memory).
Question10: What are inline functions in C, and how do they differ from macros?
Answer: Inline functions are hints to the compiler to insert the function code at the point of the function call to reduce the overhead of a function call. Unlike macros, inline functions are type-safe and are evaluated by the compiler.
Question11: What is the difference between a linker and a loader in C?
Answer: The linker combines individual object files (.o) from the compiler into a single executable. The loader loads this executable into memory for execution, resolving any dynamic linking at runtime.
Question12: How do you use the token-pasting operator (##) in C macros, and what is its purpose?
Answer: The token-pasting operator (##) in macros is used to concatenate two tokens into a single token. It's useful in macro definitions for creating variable or function names dynamically.
Question13: What is signal handling, and how can you implement a custom signal handler in C?
Answer: Signal handling allows a program to intercept and take action based on signals (interrupts). You can implement a custom signal handler using the signal() function, specifying the signal and the handler function.
Question14: What strategies can be used to avoid memory fragmentation in a C application?
Answer: To avoid memory fragmentation, use memory pools, allocate memory in fixed-size blocks, or use custom allocators that suit your application's allocation patterns.
Question15: How can you prevent dangling pointer issues in C?
Answer: To prevent dangling pointers, always set pointers to NULL after freeing the memory they point to, and be cautious with pointer arithmetic and memory reallocation.
Question16: What is the difference between #include and #import in C?
Answer: In C, #include is used to include the contents of a file or library. #import is not a standard directive in C but is used in some languages like Objective-C to ensure a file is included only once.
Question17: What is the difference between typedef and #define in C, and when should you use each?
Answer: typedef is used to create a new name (alias) for a type, while #define is used to create a macro that can represent a value or code fragment. Use typedef for creating type aliases, and #define for constants or code shortcuts.
Question18: How do you ensure proper error handling when performing file operations in C?
Answer: Check the return values of file operations (like fopen, fread, fwrite) and use feof and ferror to determine the state of the stream and handle errors appropriately.
Question19: What is the key difference between a struct and a union in C, and how does it affect memory usage?
Answer: A struct allocates separate memory for each of its members, while a union shares the same memory space among all its members, with the size equal to the size of its largest member.
Question20: What is a buffer overflow, and how can you prevent it in C?
Answer: A buffer overflow occurs when data exceeds the buffer's boundary. Prevent it by using functions that limit input size (like strncpy instead of strcpy) and by validating input length.
Full Stack Development Courses in Different Cities
- Srinagar
- Bangalore
- Gujarat
- Haryana
- Punjab
- Delhi
- Chandigarh
- Maharashtra
- Tamil Nadu
- Telangana
- Ahmedabad
- Jaipur
- Indore
- Hyderabad
- Mumbai
- Agartala
- Agra
- Allahabad
- Amritsar
- Aurangabad
- Bhopal
- Bhubaneswar
- Chennai
- Coimbatore
- Dehradun
- Dhanbad
- Dharwad
- Faridabad
- Gandhinagar
- Ghaziabad
- Gurgaon
- Guwahati
- Gwalior
- Howrah
- Jabalpur
- Jammu
- Jodhpur
- Kanpur
- Kolkata
- Kota
- Lucknow
- Ludhiana
- Noida
- Patna
- Pondicherry
- Pune