.jpg)
Abstract Class interview questions in java
Introduction:
Understanding
abstract classes is fundamental for any Java developer, especially when facing
technical interviews. Abstract classes play a crucial role in Java's object-oriented
programming, allowing developers to create a more secure, robust, and scalable
codebase. This article is tailored to help you grasp the concept of abstract classes
in Java and prepare you for related interview questions that you might encounter.
In technical interviews, questions about abstract classes are common as they test
your foundational knowledge as well as your practical experience with
object-oriented design principles. Whether you're a beginner looking to get your
feet wet or an experienced developer aiming to brush up your knowledge, the
questions and answers provided here will give you a solid understanding and
confidence to articulate your knowledge during interviews.
Dive into our comprehensive guide to not only memorize the top interview questions but also understand the underlying principles of abstract classes in Java. This will not just prepare you for your upcoming interviews but will also refine your coding practices and problem-solving approach in your daily programming tasks.
UML Diagrams of Abstract Classes and Their Subclasses:
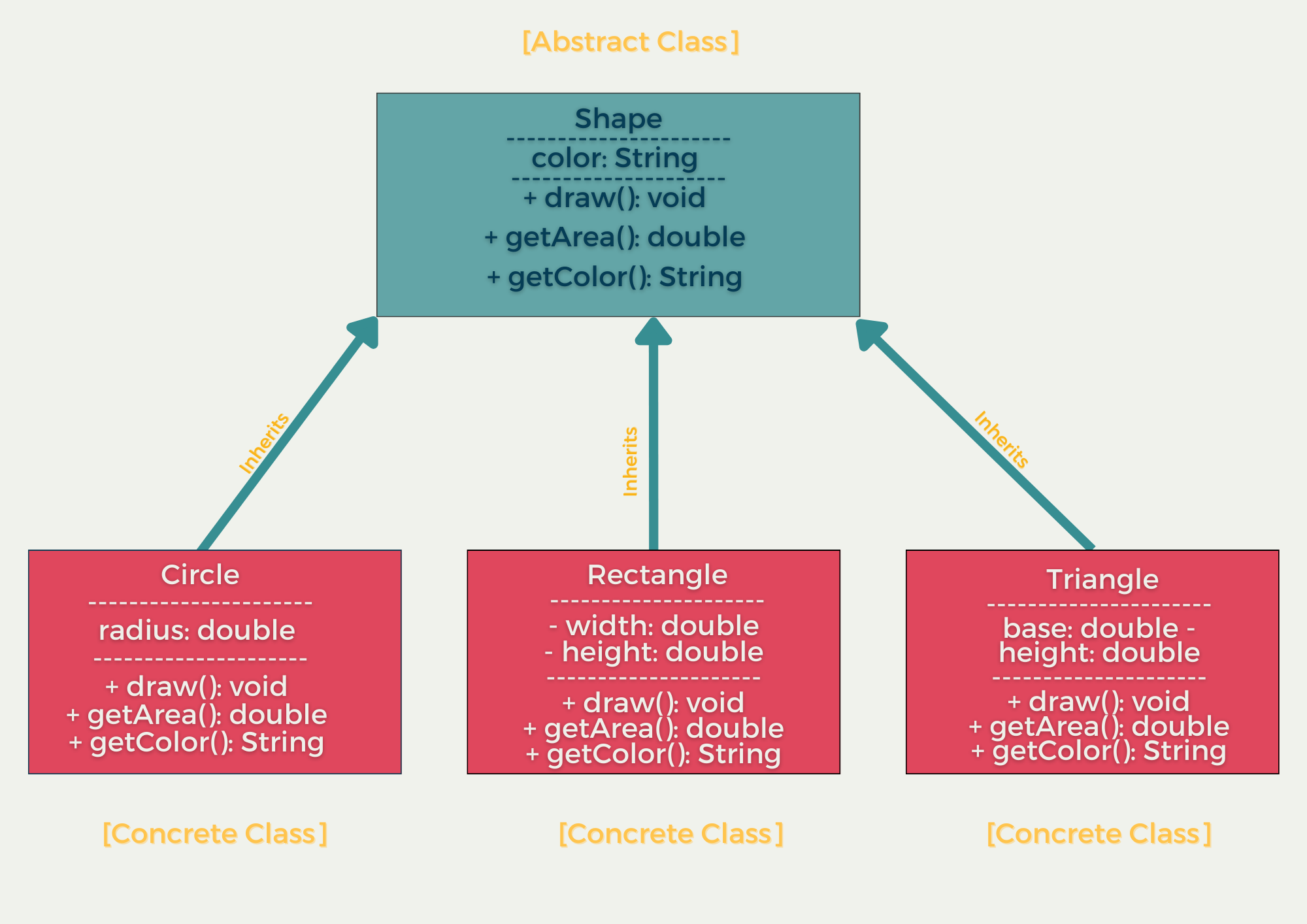
Java Abstract Class Interview Questions and Answers
1: What is an abstract class in Java?
Answer:An abstract class in Java is a superclass that cannot be instantiated and is often used to provide a base for subclasses to extend. Abstract classes are used to provide a template or design for other classes, allowing for a more structured and modular approach to programming.
2. Why can't you instantiate an abstract class in Java?
Answer: Abstract classes cannot be instantiated because they are incomplete by themselves. They may contain abstract methods that do not have a body and hence, there's no concrete implementation to invoke.
3. How does an abstract class differ from a concrete class?
Answer:An abstract class is a class that cannot be instantiated and may contain abstract methods, while a concrete class is a fully implemented class that can be instantiated. An abstract class is more of a blueprint, whereas a concrete class is a fully defined class.
4. Provide an example of an abstract class with a constructor and its purpose.
Code Example:
abstract class Vehicle {
int numberOfWheels;
Vehicle(int numberOfWheels) {
this.numberOfWheels =
numberOfWheels;
}
abstract void printType();
}
Purpose: The constructor in the abstract class 'Vehicle' allows initialization of fields (e.g., numberOfWheels) which are common to all subclasses.
5. Illustrate how to define and use an abstract method in a subclass.
Code Example:
abstract class Animal {
abstract void makeSound();
}
class Dog
extends
Animal
{
void makeSound() {
System.out.println("Bark Bark");
}
}
Usage: The abstract method makeSound is defined in the Animal class and is implemented differently in the subclass Dog.
6. Demonstrate the use of the 'abstract' keyword in declaring an abstract class and method
Code Example:
abstract class Shape {
abstract void
draw();
}
7. How do you ensure that an abstract method is overridden in all subclasses?
Code Example:
abstract class Device {
abstract void start();
}
class Phone
extends Device {
void start()
{
System.out.println("Phone is starting.");
}
}
class Laptop
extends
Device {
void start()
{
System.out.println("Laptop is starting.");
}
}
Explanation: The abstract method start in the Device class is overridden in each concrete subclass (Phone and Laptop), ensuring that each device has its specific start behavior.
8. What is the purpose of abstract methods in an abstract class?
Answer: Abstract methods act as placeholders in an abstract class. They represent methods that are common to all subclasses but require unique implementations in each subclass.
9. Demonstrate how to provide a default implementation for an abstract method in a subclass.
Code Example:
abstract class Instrument {
abstract void play();
void clean()
{
System.out.println( "Cleaning the
instrument.");
}
}
class Guitar
extends Instrument {
void play() {
System.out.println("Playing the guitar.");
}
}
10. How does an abstract class help in achieving polymorphism in Java?
Answer: Abstract classes provide a way to define methods that must be created in any subclass, thus ensuring a common interface. This allows for polymorphism, where a single interface can be used to represent different underlying forms (subclasses).
11. Show how an abstract class can provide a template method defining the structure of an algorithm.
Code Example:
abstract class CookingRecipe {
// Template method
final void prepareRecipe() {
gatherIngredients();
cook();
serve();
}
abstract void gatherIngredients();
abstract void cook();
void serve()
{
System.out.println("Serving the dish.");
}
}
12. How can you restrict a class from being subclassed in Java?
To prevent a class from being subclassed, you can declare it as final. A final class cannot be extended.
13. What are the accessibility rules for abstract methods in Java?
Abstract methods in Java must have visibility that allows them to be overridden in subclasses. They cannot be private, but they can be public or protected.
14. Show how to use abstract classes and methods to enforce certain design patterns.
Code Example:
abstract class DatabaseConnector {
abstract void connect();
void saveData(String data) {
connect();
System.out.println("Saving data: " + data);
// Implementation of saving data to the database.
}
}
class MySQLConnector extends DatabaseConnector {
void connect() {
System.out.println("Connecting to MySQL
database.");
}
}
15.What happens if a subclass of an abstract class does not implement all the abstract methods?
Answer: If a subclass does not implement all the abstract methods, it must also be declared abstract. Otherwise, the code will not compile.
16.Demonstrate how polymorphism works with abstract classes and methods.
Code Example:
abstract class Payment {
abstract void pay();
}
class CreditCardPayment extends Payment
{
void pay() {
System.out.println("Paying with Credit
Card.");
}
}
class PayPalPayment extends Payment
{
void pay()
{
System.out.println("Paying with
PayPal.");
}
}
17.How does an abstract class differ from an interface in Java?
Answer: An abstract class can have instance methods that implement a default behavior, while an interface can only declare methods and properties but not implement them (until Java 8, where default and static methods in interfaces were introduced).
18. Demonstrate how an abstract class can implement an interface without providing an implementation for all of its methods.
Code Example:
interface Movable {
void move();
}
abstract class Vehicle implements Movable {
// No implementation for move, letting the concrete subclass provide it.
}
class Car
extends
Vehicle {
void move()
{
System.out.println("Car is moving.");
}
}
19. Show how an abstract class and interface can be used together to provide a flexible design.
Code Example:
interface Chargeable {
void charge();
}
abstract class ElectronicDevice implements Chargeable {
abstract void use();
}
class Smartphone
extends
ElectronicDevice {
void use()
{
System.out.println("Using smartphone.");
}
public void charge() {
System.out.println("Charging
smartphone.");
}
}
20. What are some best practices when using abstract classes in Java?
Answer: Use abstract classes when you want to share code among several closely related classes,
Full Stack Development Courses in Different Cities
- Srinagar
- Bangalore
- Gujarat
- Haryana
- Punjab
- Delhi
- Chandigarh
- Maharashtra
- Tamil Nadu
- Telangana
- Ahmedabad
- Jaipur
- Indore
- Hyderabad
- Mumbai
- Agartala
- Agra
- Allahabad
- Amritsar
- Aurangabad
- Bhopal
- Bhubaneswar
- Chennai
- Coimbatore
- Dehradun
- Dhanbad
- Dharwad
- Faridabad
- Gandhinagar
- Ghaziabad
- Gurgaon
- Guwahati
- Gwalior
- Howrah
- Jabalpur
- Jammu
- Jodhpur
- Kanpur
- Kolkata
- Kota
- Lucknow
- Ludhiana
- Noida
- Patna
- Pondicherry
- Pune